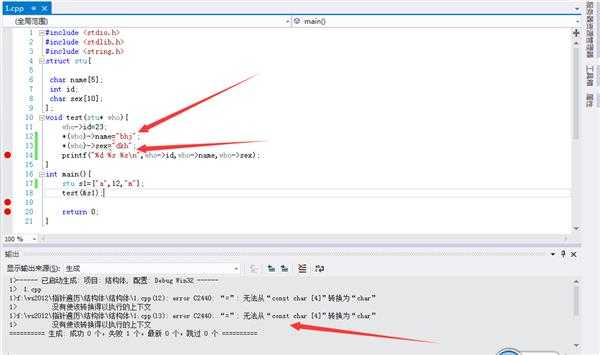
#include <stdlib.h>
#include <string.h>
struct stu{
char name[5];
int id;
char sex[10];
};
void test(stu* who){
who->id=23;
*(who)->name=”bhj”;
*(who)->sex=”dkh”;
printf(“%d %s %s\n”,who->id,who->name,who->sex);
}
int main(){
stu s1={“a”,12,”m”};
test(&s1);
return 0;
}
可以帮本人解答一下吗?谢谢高手了
解决方案
10
#include <stdio.h> #include <stdlib.h> #include <string.h> struct stu{ char name[5]; int id; char sex[10]; }; void test(struct stu *who){ who->id=23; who->name[0]="b"; who->name[1]="h"; who->name[2]="j"; who->sex[0]="d"; who->sex[1]="k"; who->sex[2]="h"; printf("%d %s %s\n",who->id,who->name,who->sex); } int main(){ stu s1={"a",12,"m"}; test(&s1); return 0; }
不知道你想要干嘛,只是改的没错了
10
*(who)->
这个代码是什么?
->操作符 的操作对象本身就是对结构体指针,你加个*以后,就是这个结构体的第一个元素的地址的内容了
也就是name[0]了(可能说得不大准确,大致就是这个意思了),反正就是语法已经乱了
所以才会报错“无法从 const char [4] 转换成 char”
20
字符串拷贝用strcpy
#include <stdio.h> #include <stdlib.h> #include <string.h> struct stu{ char name[5]; int id; char sex[10]; }; void test(stu* who){ who->id=23; strcpy(who->name, "bhj"); strcpy(who->sex, "dkh"); printf("%d %s %s\n",who->id,who->name,who->sex); } int main(){ stu s1={"a",12,"m"}; test(&s1); return 0; }
10
哦 把字符当作字符串输出,是严重的错误
*(who)-> name ////这里的 优先级运算符 () 不起任何作用,纯属骗人的 ===>* who -> name ==>*(who->name)