# include <stdio.h>
# include <stdlib.h>
# include <malloc.h>
struct NODE
{
int data;
struct NODE *pNext;
};
struct POINT
{
struct NODE *Top;
struct NODE *Bottom;
};
struct POINT *init(void);
void push(struct POINT *S);
void traverse(struct POINT *S);
int main()
{
struct POINT *S = NULL;
int n;
S = init();
if (S != NULL)
printf(“栈 初始化成功\n”);
printf(“请输入你需要的结点数: “);
scanf(“%d”, &n);
while (n–)
{
push(S);
}
traverse(S);
return 0;
}
struct POINT *init(void)
{
struct POINT *q = (struct POINT *)malloc(sizeof(struct POINT));
if (q == NULL)
{
printf(“内存分配失败\n”);
exit(-1);
}
struct NODE *p = (struct NODE *)malloc(sizeof(struct NODE));
if (p == NULL)
{
printf(“内存分配失败\n”);
exit(-1);
}
q->Top = p;
q->Bottom = p;
p->pNext = NULL;
}
void push(struct POINT *S)
{
int val;
printf(“请输入你需要压栈的值 “);
scanf(“%d”, &val);
struct NODE *p = (struct NODE *)malloc(sizeof(struct NODE));
if (p == NULL)
{
printf(“内存分配失败\n”);
exit(-1);
}
p->data = val;
p->pNext = S->Top;
S->Top = p;
}
void traverse(struct POINT *S)
{
struct NODE *p = S->Top;
while (p != S->Bottom)
{
printf(“%d “, p->data);
p = p->pNext;
}
printf(“\n”);
}
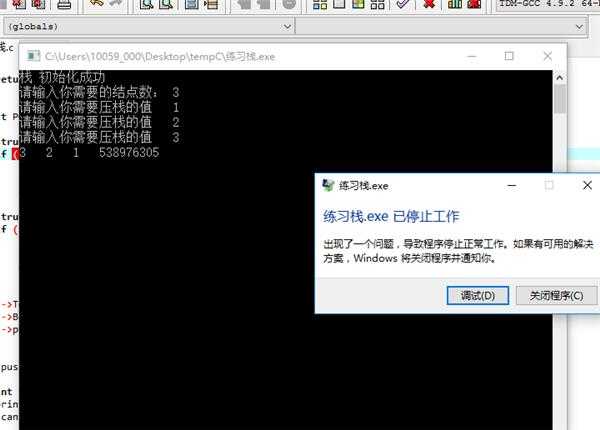
# include <stdlib.h>
# include <malloc.h>
struct NODE
{
int data;
struct NODE *pNext;
};
struct POINT
{
struct NODE *Top;
struct NODE *Bottom;
};
struct POINT *init(void);
void push(struct POINT *S);
void traverse(struct POINT *S);
int main()
{
struct POINT *S = NULL;
int n;
S = init();
if (S != NULL)
printf(“栈 初始化成功\n”);
printf(“请输入你需要的结点数: “);
scanf(“%d”, &n);
while (n–)
{
push(S);
}
traverse(S);
return 0;
}
struct POINT *init(void)
{
struct POINT *q = (struct POINT *)malloc(sizeof(struct POINT));
if (q == NULL)
{
printf(“内存分配失败\n”);
exit(-1);
}
struct NODE *p = (struct NODE *)malloc(sizeof(struct NODE));
if (p == NULL)
{
printf(“内存分配失败\n”);
exit(-1);
}
q->Top = p;
q->Bottom = p;
p->pNext = NULL;
}
void push(struct POINT *S)
{
int val;
printf(“请输入你需要压栈的值 “);
scanf(“%d”, &val);
struct NODE *p = (struct NODE *)malloc(sizeof(struct NODE));
if (p == NULL)
{
printf(“内存分配失败\n”);
exit(-1);
}
p->data = val;
p->pNext = S->Top;
S->Top = p;
}
void traverse(struct POINT *S)
{
struct NODE *p = S->Top;
while (p != S->Bottom)
{
printf(“%d “, p->data);
p = p->pNext;
}
printf(“\n”);
}
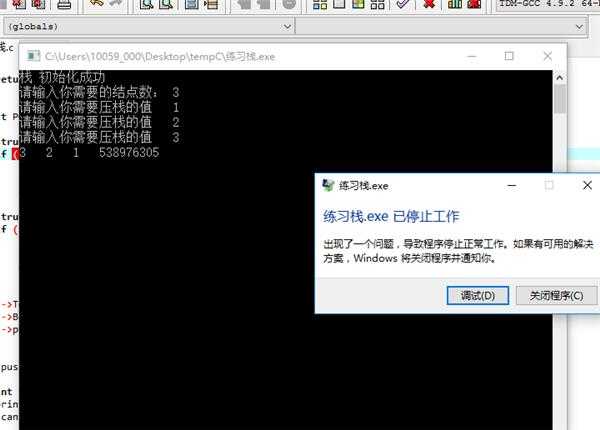
解决方案
5
main.c(61): warning C4715: “init”: 不是全部的控件路径都返回值
30
1.你的init函数都没有return q;
2.你的init函数里malloc出来的p感觉也没啥意义
3.在你的push函数里加了一步if (S->Bottom == NULL) S->Bottom = p;
4.traverse里修改了下while的条件
2.你的init函数里malloc出来的p感觉也没啥意义
3.在你的push函数里加了一步if (S->Bottom == NULL) S->Bottom = p;
4.traverse里修改了下while的条件
# include <stdio.h> # include <stdlib.h> # include <malloc.h> struct NODE { int data; struct NODE *pNext; }; struct POINT { struct NODE *Top; struct NODE *Bottom; }; struct POINT *init(void); void push(struct POINT *S); void traverse(struct POINT *S); int main() { struct POINT *S = NULL; int n; S = init(); if (S != NULL) printf("栈 初始化成功\n"); printf("请输入你需要的结点数: "); scanf("%d", &n); while (n--) { push(S); } traverse(S); return 0; } struct POINT *init(void) { struct POINT *q = (struct POINT *)malloc(sizeof(struct POINT)); if (q == NULL) { printf("内存分配失败\n"); exit(-1); } q->Top = NULL; q->Bottom = NULL; return q; } void push(struct POINT *S) { int val; printf("请输入你需要压栈的值 "); scanf("%d", &val); struct NODE *p = (struct NODE *)malloc(sizeof(struct NODE)); if (p == NULL) { printf("内存分配失败\n"); exit(-1); } if (S->Bottom == NULL) S->Bottom = p; p->data = val; p->pNext = S->Top; S->Top = p; } void traverse(struct POINT *S) { struct NODE *p = S->Top; while (p) { printf("%d ", p->data); p = p->pNext; } printf("\n"); }