#include<iostream.h>
#include<string>
class Str
{
int length;
char *p;
public:
Str()
{
p=new char[100];
}
Str(char *s)
{
p=new char [100];
strcpy(p,s);
}
Str(const Str &obj)
{
p=obj.getp();
}
~Str()
{
delete []p;
}
friend Str operator+(const Str &s1,const Str &s2);
void operator =(const Str &obj);
char* getp()const
{
return p;
}
void setp(char *s)
{
strcpy(p,s);
}
void show()
{
length=strlen(p);
cout<<“length=”<<length<<” “<<p<<endl;
}
};
Str operator + (const Str &s1,const Str &s2) //报错处
{
Str temp;
strcpy(temp.getp(),s1.getp());
strcat(temp.getp(),s2.getp());
return temp;
}
void Str::operator =(const Str &obj)
{
strcpy(p,obj.getp());
}
int main()
{
char s1[100],s2[100];
cin.getline(s1,100);
cin.getline(s2,100);
Str A(s1);
Str B(s2);
Str C;
C=A+B;
C.show();
return 0;
}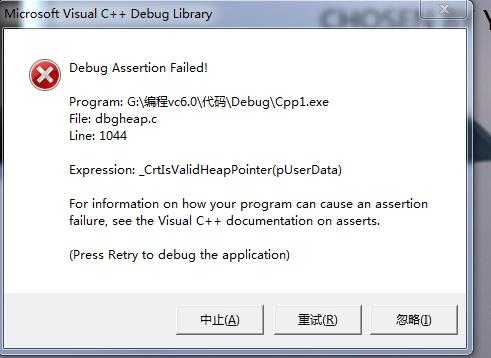
#include<string>
class Str
{
int length;
char *p;
public:
Str()
{
p=new char[100];
}
Str(char *s)
{
p=new char [100];
strcpy(p,s);
}
Str(const Str &obj)
{
p=obj.getp();
}
~Str()
{
delete []p;
}
friend Str operator+(const Str &s1,const Str &s2);
void operator =(const Str &obj);
char* getp()const
{
return p;
}
void setp(char *s)
{
strcpy(p,s);
}
void show()
{
length=strlen(p);
cout<<“length=”<<length<<” “<<p<<endl;
}
};
Str operator + (const Str &s1,const Str &s2) //报错处
{
Str temp;
strcpy(temp.getp(),s1.getp());
strcat(temp.getp(),s2.getp());
return temp;
}
void Str::operator =(const Str &obj)
{
strcpy(p,obj.getp());
}
int main()
{
char s1[100],s2[100];
cin.getline(s1,100);
cin.getline(s2,100);
Str A(s1);
Str B(s2);
Str C;
C=A+B;
C.show();
return 0;
}
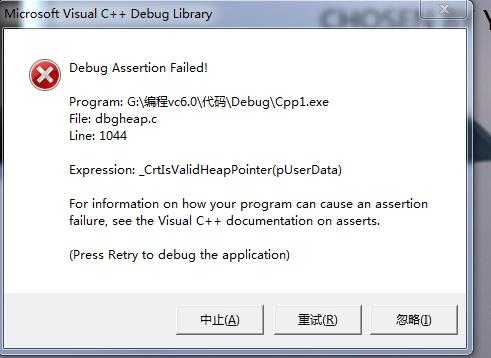
解决方案
3
拷贝构造函数写的不对。得new出字符串 之后再strcpy
16
拷贝构造里没有给p分配空间,导致析构出错
PS:其实假如改成release的话(编译器优化过的话是不会调用拷贝构造的,也就不会报错)
PS:其实假如改成release的话(编译器优化过的话是不会调用拷贝构造的,也就不会报错)
#include<iostream> #include<string> using namespace std; class Str { int length; char *p; public: Str() { p=new char[100]; } Str(char *s) { p=new char [100]; strcpy(p,s); } Str(const Str &obj) { p=new char [100]; strcpy(p,obj.getp()); } ~Str() { delete []p; } friend Str operator+(const Str &s1,const Str &s2); void operator =(const Str &obj); char* getp()const { return p; } void setp(char *s) { strcpy(p,s); } void show() { length=strlen(p); cout<<"length="<<length<<" "<<p<<endl; } }; Str operator + (const Str &s1,const Str &s2) //报错处 { Str temp; strcpy(temp.getp(),s1.getp()); strcat(temp.getp(),s2.getp()); return temp; } void Str::operator =(const Str &obj) { strcpy(p,obj.getp()); } int main() { char s1[100],s2[100]; cin.getline(s1,100); cin.getline(s2,100); Str A(s1); Str B(s2); Str C; C=A+B; C.show(); return 0; }
18
构造函数是构造一个新的对象,你之前分配的内存是属于原对象,故你需要重新分配内存