最近在看《Visual C++2013入门经典》,看完第9章,做后面的习题,第4题。题目要求定义一个存储整数值的有序二叉树BinaryTree,节点类Node作为该类的内部类。然后编写一个程序,存储任意顺序的整数,然后以升序的方式检索并输出树中存储的这些整数值。编译环境VS2013,然后本人本人实现的简单代码如下:
(1)BinaryTree.h
(1)BinaryTree.h
#pragma once class CBinaryTree { public: CBinaryTree(); CBinaryTree(int a[], size_t count); CBinaryTree(const CBinaryTree& bt) = delete; CBinaryTree& operator=(const CBinaryTree& bt) = delete; ~CBinaryTree(); void add(int val); // add a new val void print() const; // List all the value in ascending order private: struct Node { int value; // node value Node* pn_left; // left node pointer Node* pn_right; // right node pointer // constructor Node(int val, Node* pnL=nullptr, Node* pnR=nullptr) :value{ val }, pn_left{ pnL }, pn_right{pnR} {} }; Node* m_pRoot{}; private: void insert(Node** ppnCurRoot, Node* pnTarget); // insert a node to the tree using recurrence void remove(Node** ppnCurRoot); void travel(Node* pnRoot) const; // travel sub tree using recurrence };
(2)BinaryTree.cpp
#include "BinaryTree.h" #include <iostream> CBinaryTree::CBinaryTree() { } CBinaryTree::CBinaryTree(int a[], size_t count) { for (size_t i{}; i < count; i++) add(a[i]); } CBinaryTree::~CBinaryTree() { remove(&m_pRoot); } // add a new val void CBinaryTree::add(int val) { Node* pn = new Node{ val }; insert(&m_pRoot, pn); } // List all the value in ascending order void CBinaryTree::print() const { std::cout << "BinaryTree datas as below:" << std::endl; travel(m_pRoot); std::cout << std::endl; } // insert a node to the tree using recurrence void CBinaryTree::insert(Node** ppnCurRoot, Node* pnTarget) { Node* pnCurRoot{ *ppnCurRoot }; // pnCurRoot: current root node pointer value if (!pnCurRoot) { *ppnCurRoot = pnTarget; return; } if (pnTarget->value <= pnCurRoot->value) insert(&pnCurRoot->pn_left, pnTarget); else insert(&pnCurRoot->pn_right, pnTarget); } void CBinaryTree::remove(Node** ppnCurRoot) { Node* pnCurRoot{ *ppnCurRoot }; if (!pnCurRoot) return; remove(&pnCurRoot->pn_left); // remove left-sub tree of current root node pnCurRoot->pn_left = nullptr; remove(&pnCurRoot->pn_right); // remove right-sub tree of current root node pnCurRoot->pn_right = nullptr; delete pnCurRoot; // remove current root node pnCurRoot = nullptr; } // travel sub tree using recurrence void CBinaryTree::travel(Node* pnRoot) const { if (!pnRoot) return; travel(pnRoot->pn_left); std::cout << pnRoot->value << " "; travel(pnRoot->pn_right); }
(3)main.cpp
#include "BinaryTree.h" #include <cstdlib> int main() { int values[]{-1, 5, 3, 8, 2, -4, 9, 7, 11, 22, -5}; CBinaryTree bt{ values, _countof(values) }; bt.print(); return 0; }
然后编译通过,运行结果也正常。但是本人在main中CBinaryTree bt{ values, _countof(values) };这句前面加断点,然后以单步F11调试运行时,发现一步步按F11,过一会就弹出下图所示的“未找到stack.cpp”,从没碰到过这种情况,新手讨教一下,这是怎么回事啊?麻烦帮看一下,谢谢!
解决方案
4
这样改了试下:项目属性——C/C++——代码生成——运行库:Debug用MTd Release用MT
4
假如你在进行C语言的编程的话最好避开c++的编译器 否则就有可能造成这种错误。
也可能是 “Enable .NET Framework source stepping”
看看这个:http://stackoverflow.com/questions/22239324/stack-cpp-not-found-prevents-debug-in-vs-2013-express
也可能是 “Enable .NET Framework source stepping”
看看这个:http://stackoverflow.com/questions/22239324/stack-cpp-not-found-prevents-debug-in-vs-2013-express
1
假如某些操作调用了系统函数,那么没有源码是很正常的
不用介意
不用介意
15
本人模拟试了一下题主的代码
原因是题主的代码里,递归结束的时候,编译器插入了__rtc_checkesp函数来动态监测程序能否运行正常,而这个函数是系统提供的,实现在stack.cpp里,题主又没有源码,所以就弹提示了
这种东西不必介意,不是题主的问题
想不出现的话,就按下图设置圈圈处的东西,估计题主原本设的是both或Stack Frames(主要就是这个)
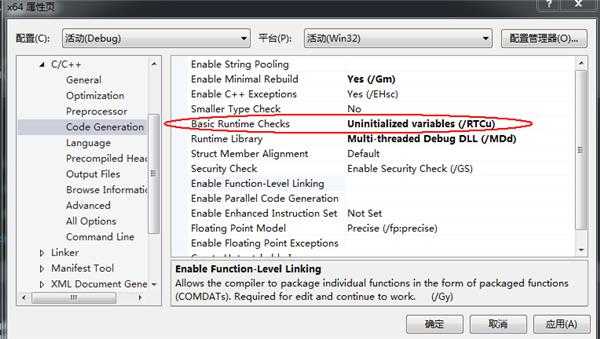
原因是题主的代码里,递归结束的时候,编译器插入了__rtc_checkesp函数来动态监测程序能否运行正常,而这个函数是系统提供的,实现在stack.cpp里,题主又没有源码,所以就弹提示了
这种东西不必介意,不是题主的问题
想不出现的话,就按下图设置圈圈处的东西,估计题主原本设的是both或Stack Frames(主要就是这个)
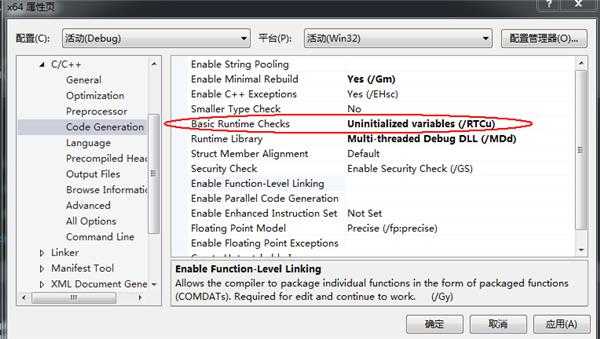